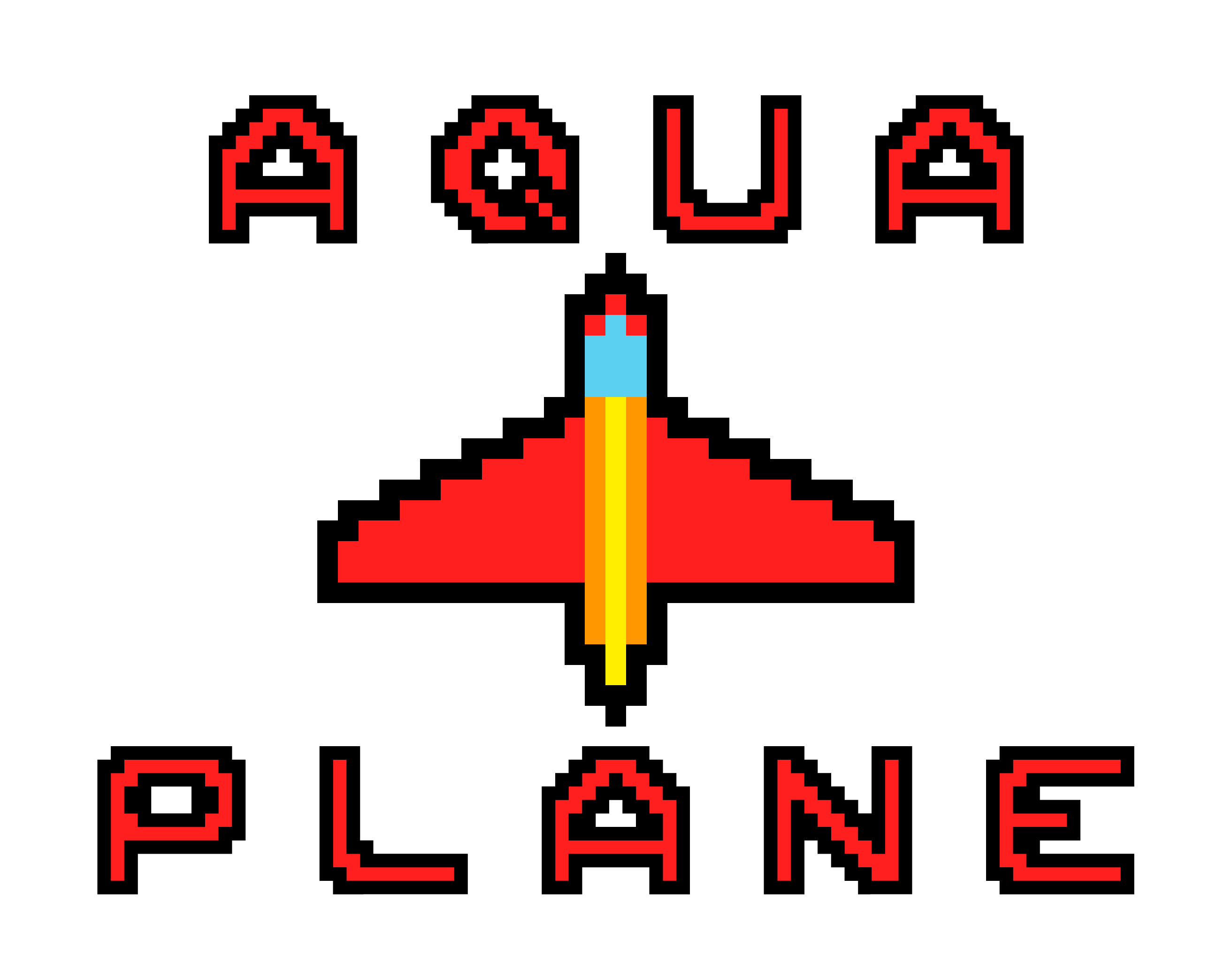
Aqua Plane
Aqua Plane is a classic top-down shooter.
Initial Design Plans
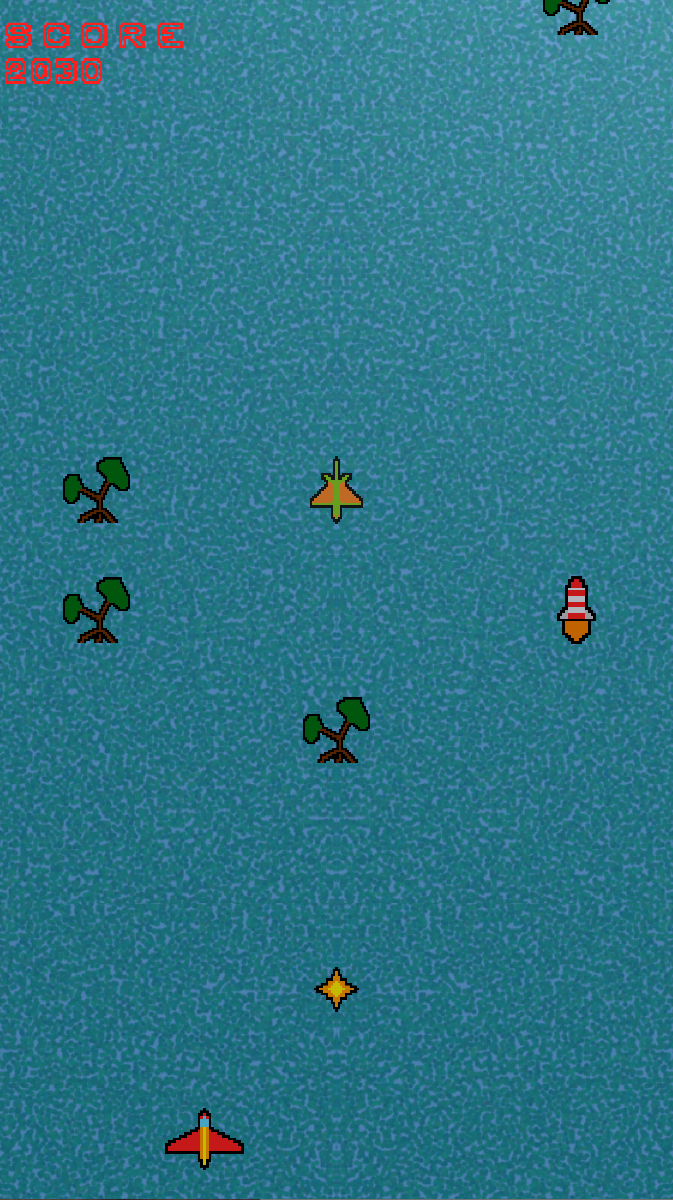
The original limitation that I put upon this game was that everything had to die in one hit. The player, the enemies, and even the bosses -- everything had to be a glass cannon. With this in mind, I thought that the genre that would work best with this limitation would be a top-down shooter, as there are many games in the bullet-hell genre that work with this assumption already. This also influenced my decision to use pixel art. Since it's an older-style game, and older art style would help to drive the point home further.
Enemy/Boss Design
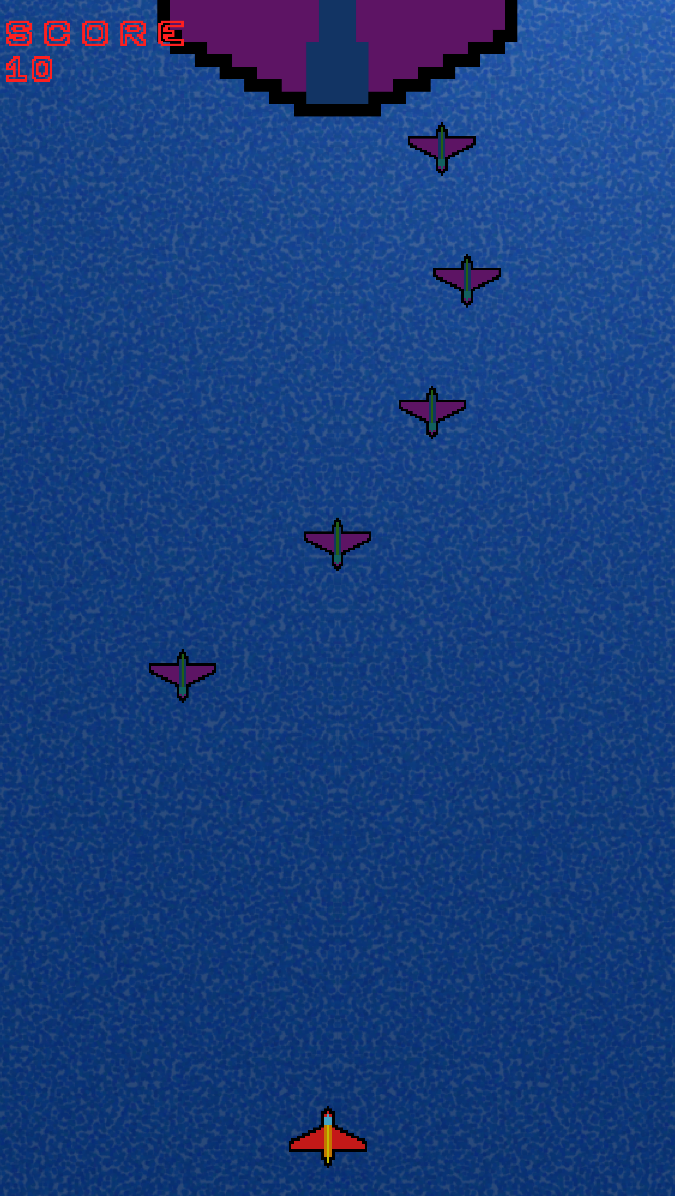
Since the player always dies in one hit, I had to design each boss and enemy so that they wouldn't be too punishing but still put up a decent challenge. Especially with the bosses, I tried to incentivise cleverness over brute-force, as many of the bosses can be beaten quite easily with a well-timed rocket.
Enemy Spawning
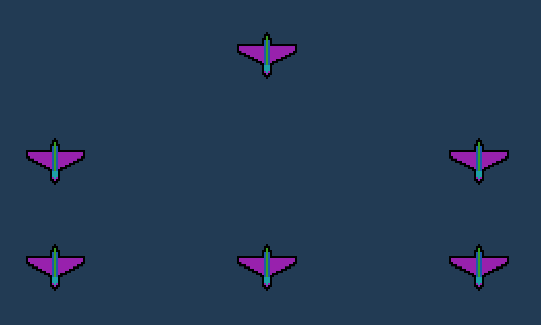
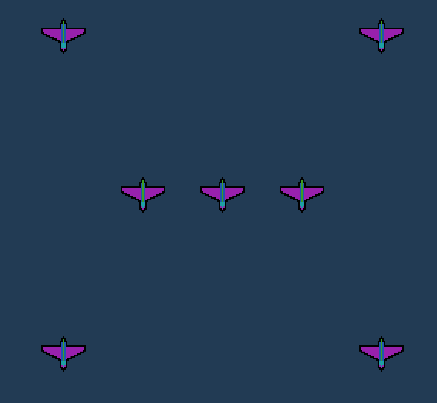
I wanted to make the enemies spawn randomly, but still using patterns, in the fashion that old space shooters tended to do. So instead of choosing random enemies to spawn, the game chooses random preset patterns, which allows for the gameplay to be more dynamic without becoming unfair by spawning an impassable wave of enemies.
Spawning Snippet
void FixedUpdate() { waitingTime -= Time.deltaTime; if (waitingTime <= 0 && !bossFight) { GameObject formation; formationNumber = Random.Range(0, numberOfFormations); formation = Instantiate(formationArray[formationNumber], this.transform.position, Quaternion.Euler(0, 0, 0)); numberOfChildren = formation.transform.childCount; numberToDelete = Random.Range(1, numberOfChildren); //always delete at least one child, so that the player has a path through. for (int i = 0; i < numberToDelete; i++) { childToDelete = Random.Range(0, numberOfChildren-deletedChildren); if (PlayerControls.hasMissile == false && Random.Range(0.0f, 100.0f) <= 10.0f && !missileInFormation) //chance to replace a deleted child with a missile, if the player doesn't have a missile. { missileInFormation = true; GameObject newMissile; newMissile = Instantiate(MissilePowerup, formation.transform.GetChild(childToDelete).position, Quaternion.Euler(0, 0, 0)); } else if (numberToDelete > 1 && alternativeSpawn != null && Random.Range(0.0f, 100.0f) <= 10.0f) //chance to replace an extra deleted child with a special enemy { GameObject newSpawn; newSpawn = Instantiate(alternativeSpawn, formation.transform.GetChild(childToDelete).position, Quaternion.Euler(0, 0, 0)); } Destroy(formation.transform.GetChild(childToDelete).gameObject); deletedChildren++; } if (spawningInterval > 2f && levelPhase == 1 || spawningInterval > 1f && levelPhase == 2) //spawn formations faster and faster as time goes on. { spawningInterval -= 0.25f; } else //after the spawning interval reaches the minimum time, keep spawning formations at this now static spawning interval for a set amount of "rounds". { rounds++; } waitingTime = spawningInterval; deletedChildren = 0; missileInFormation = false; } if (rounds >= maxRounds) //once all spawning rounds are complete, stop spawning formations, spawn the boss, and destroy this script. { rounds = 0; bossFight = true; Boss.SetActive(true); Destroy(this); } }
UI and Graphics
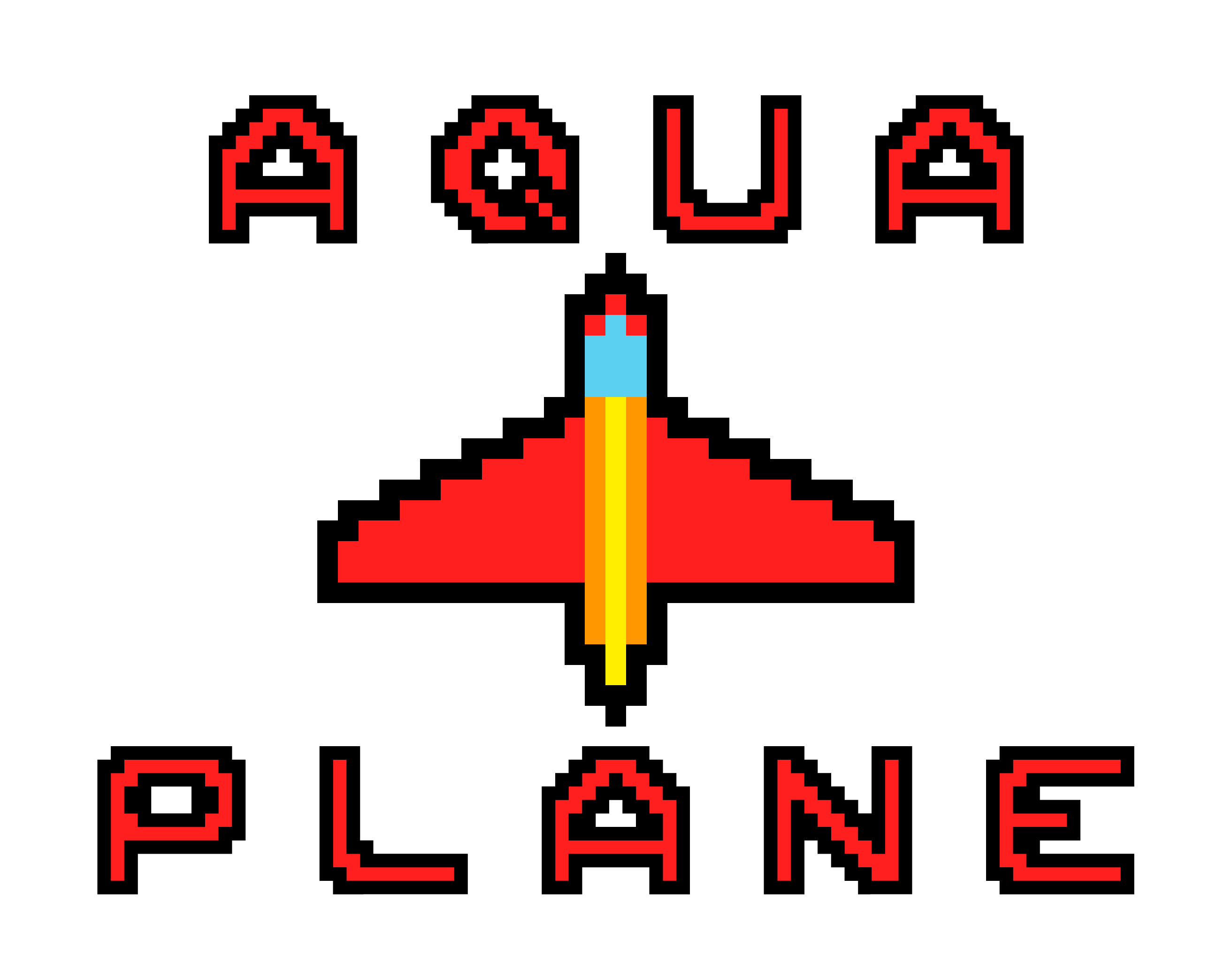

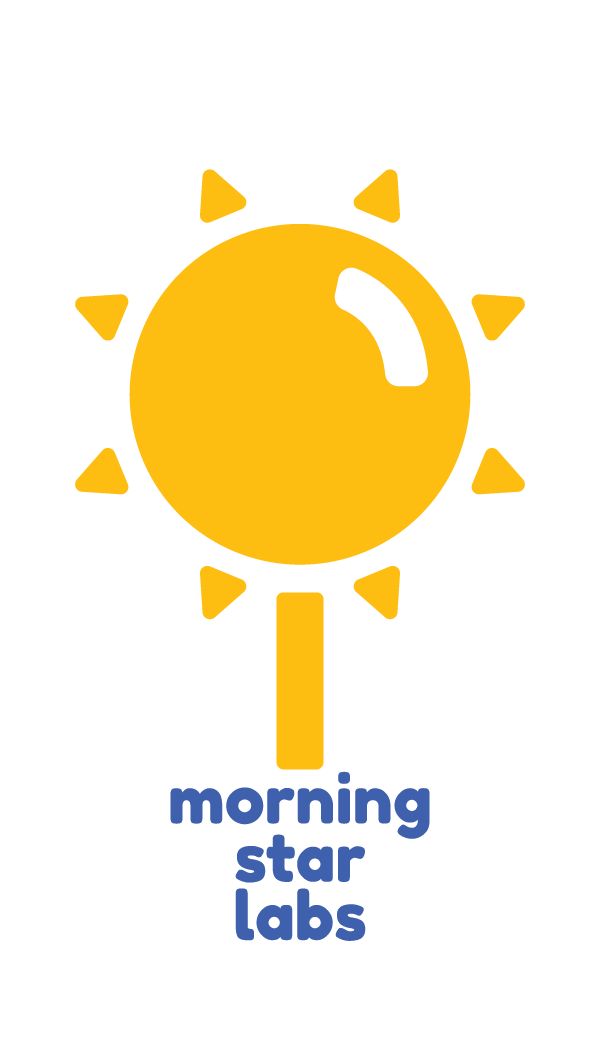
This is the first time I ever did spritework, and although it was challenging at some points, it was also quite fun. I also went through the process of making a font for personal use, tentatively called "AquaBits", for the purpose of writing out titles and words on buttons easier. Finally, I felt I had to come up with some sort of title to go under for games that were made by me personally, as such "Morning Star Labs" was born, along with an accompanying logo.
Music and Sound Effects
The music was made in MuseScore 3, and the sound effects were made in bfxr, which is an old but gold program for making 8-bit sounds.